WordPress developers aren’t famous for their code quality and we’re all paying for it!
The extensibility of WordPress and low barrier of entry for developers to build with it have led to the mass adoption we see today. What we don’t see when we visit a typical WordPress site, is the low quality code in some of the 50+ installed plugins or the site’s theme. We don’t see it, but we can often feel it in slow loading or buggy pages. The site owner may feel it in their wallet, paying for more powerful servers to run their increasingly bloated sites.
That’s just poorly optimised code causing performance issues, but let’s not omit talking about the security issues that poor quality code brings! If you’re a developer submitting a plugin to wp.org for the first time, the code will be reviewed by the plugin team and obvious issues flagged for correction. Once approved though, there isn’t a lot in place to prevent you from introducing security issues in your next release and less to warn you about performance issues or broken functionality. And what noble, but small measures are in place are only for wp.org listed plugins and can’t check premium plugin code or those, like my WP2Static, not listed in the main WordPress plugin repository.
WP Owls already mitigates these performance and security issues by publishing their site statically, using WP2Static. Pre-rendering your site to static this way can mitigate many performance issues due to poorly coded plugins/themes. Any security issues in WordPress core or plugins/themes are completely removed from the site served to the public with this setup, too.
Going static isn’t a silver bullet to WordPress code quality issues, though. If you’re trying to publish a 100,000 page site and each page in WordPress takes 3 seconds to load due to poor performance, that’s going to greatly slow down your static site publishing process. Other sites, such as those relying on WooCommerce, won’t be easy to switch to static publishing today.
So, these code quality problems can’t always be ignored. WordPress can’t currently detect many issues with any plugins/themes you install. There is the official Tide project, from the WordPress team, which aims to “run automated quality testing for all WordPress plugins and themes and make those test results visible for both the authors and the end users of those plugins and themes”, but it’s limited in what it scans for and again, won’t be able to touch the premium plugins or those not on wp.org.
It is thus, up to us, as WordPress developers, to adopt code quality measures in our plugins and themes. Not only for the great performance and security benefits it provides our users, but by also catching bugs early in development. This gives us confidence that our software behaves as we expect. So, we’ll have less support requests, more sales and a better night’s sleep!
Why you should subscribe to our newsletter?
✔️ Learn from WP experts
✔️ Be up to date with what is happening in WordPress space
✔️ No spam
PHP CodeSniffer
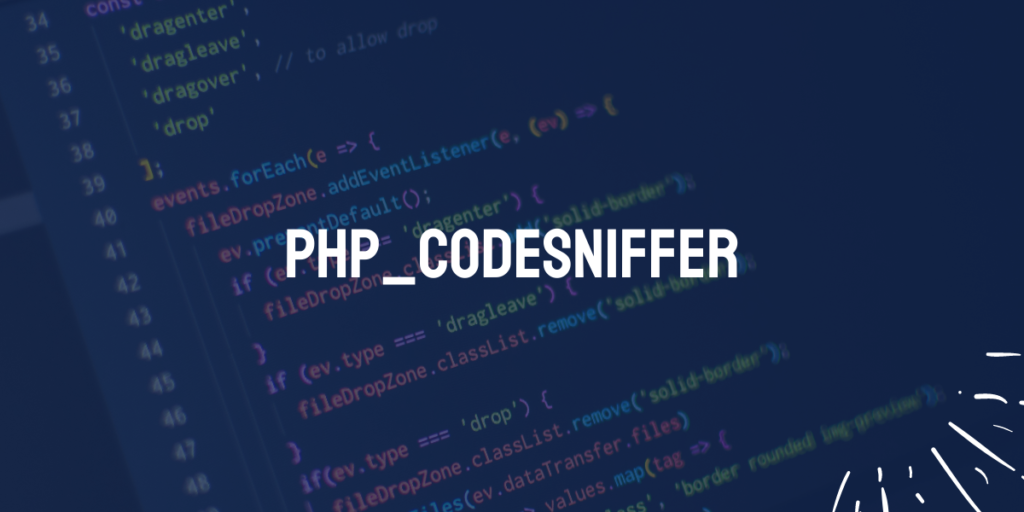
I am not what I’d call a professional programmer. Impostor syndrome aside, I’ve read like 1 and a half books on programming, don’t watch videos and it’s been years since I read many tutorials. I somehow manage to create functional software, using some basic knowledge from years ago, skimming official docs and relying too much on my strengths in troubleshooting and willingness to keep adjusting things until they work. My brain also hurts with complexity, so I try and write code simple enough for me understand. Anyway, for years, I got by, as most WordPress developers do.
In 2018, though, my world was shaken up by two well-intentioned developers, who wanted to contribute to my open source project, WP2Static. I love it when contributors come and deliver you a whole new feature or fix a bug in the project. I can just review it, merge it in and all users benefit without me having to use my brain much. But, not in this case! These contributors wanted me to change the way I develop my software! Gray Arswright and Viktor Szepe both approached me, not only encouraging me to adopt PHP Code Sniffer (PHPCS) rules and tooling in my project, but even offering to do most of the initial work to get it running and code tests passing.
I declined them. I was stubborn. I didn’t want to change. This new tooling was going to affect every file in my project and I had to now fix a bunch of supposed errors I’d been oblivious to? Luckily, their egos were better than mine and they persisted to convince me of the benefits of adopting code quality tooling and I’ve never looked back since! To this day, PHPCS helps me to develop faster and catch many simple code issues early. My sincere thanks, Gray and Viktor!
PHP Code Sniffer can be run manually, in a build process or automatically within your code editor to alert you of coding standard violations. There are a bunch of popular coding standard rulesets defined which you can choose to adhere to, such as the official WordPress Coding Standards. I prefer the standard in Viktor’s PSR 12 Neutron Hybrid Ruleset. This is based off Automattic’s Neutron Ruleset, for more modern WordPress code. As WordPress core chooses to still support PHP versions as old as 5.6.2 (as at WordPress 5.8), the WordPress Coding Standards aren’t great for modern PHP code. You can choose to override specific rules from a ruleset, ie, if you’re using WordPress Coding Standards, which force the old array() syntax, but your team really prefers the modern short array syntax of [], you can make that override. If submitting your plugin to wp.org though, you may need to adhere to the more legacy coding standards.
A huge thanks to Greg Sherwood, the lead developer of PHPCS, along with all the project’s contributors!
PHPStan
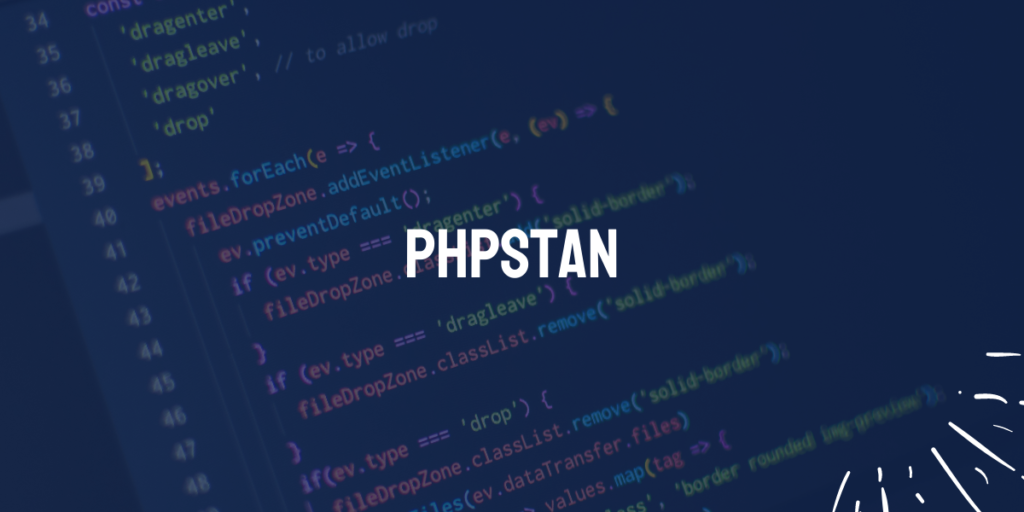
I also need to thank Viktor, for getting me started with this powerful static analysis tool and continuing to help me when I get stuck!
Where PHPCS analyses each file individually, for obvious syntactical issues, PHPStan goes much deeper. PHPStan will load your classes and report things like namespace violations, trying to call functions with incorrect arguments and many more useful tests. PHPStan can be run with varying levels of strictness. While I started with something midway, I almost immediately set it to the strictest checking – I mean, who wants OK quality when you can have great quality, amirite? PHPStan will look at your PHPDocs to see what a function’s argument type is, then alert you if anywhere in your code, you could possibly call it with an unsupported type of variable. This is so powerful and can shut down a whole range of bugs and security issues before they have a chance to do damage! We’re seeing more native type hints coming with each new PHP version, but this doesn’t lessen all the other value PHPStan provides and many projects will still need to rely on PHPDocs until they can stop supporting older PHP versions.
Big thanks to Ondřej Mirtes for all the work he does with PHPStan, along with all the project’s other contributors. Ondřej also released PHPStan Pro last year, which I’m sure many will find handy additions to their workflows. Also more thanks to Viktor for maintaining the PHPStan WordPress extensions!
I’m yet to start using the Psalm code quality tool, it seems many start with either PHPStan or Psalm. I’d love to run both, I have just been lazy. Many users say there is a use case for running both, so please enjoy trying them both out!
PHPUnit
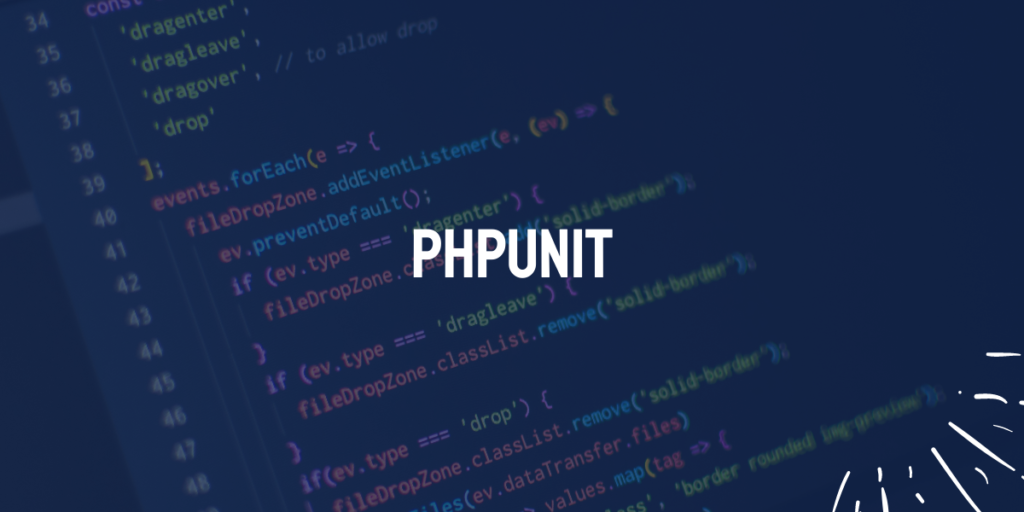
There are so many levels of code quality tooling, from the simple linter built into PHP (php -l filename.php) through to mutation testing frameworks, like Infection.
I can’t cover them all in this article, but I want to include PHPUnit. With just PHP Code Sniffer, PHPStan and PHPUnit integrated to your WordPress project, you’ll gain an amazing amount of confidence in your project’s quality.
PHPUnit is a unit testing framework, which, like many others, derives structure and behaviour from the Smalltalk languages’s SUnit framework (TIL!). Unlike PHPCS and PHPStan, you can’t just install it, set some config options and immediately benefit from it telling you all the coding mistakes you’ve made. With PHPUnit, you’ll need to write your own tests. Yeah, so the least appealing to the lazy developer in us!
Once you get started with unit testing though, you won’t want to write code without it. Or you will, then curse yourself when a bug is reported that you know you could have avoided, had you written unit tests!
PHPUnit isn’t limited to unit tests, you can also use it to run your integration and end to end tests, too. Writing automated software tests is a whole other topic that you can research. My advice is to also run a code coverage tool (such as provided by XDebug), which will not only give you nice feedback about what % of your code is covered by unit tests, but also general indicators of overly complex or risky code you should address next.
Much appreciation to Sebastian Bergmann, Andreas Möller and all the contributors to PHPUnit!
Outro
There’s a world of amazing code quality tooling out there for PHP and WordPress today. Please don’t be stubborn, as I initially was. Adopt code quality tooling into your projects and we’ll all sleep better at night!
If you have questions or need help getting started adopting these tools, I’ll do my best to help. @ me in your GitHub project or email me@ljs.dev